Android Development with Kotlin
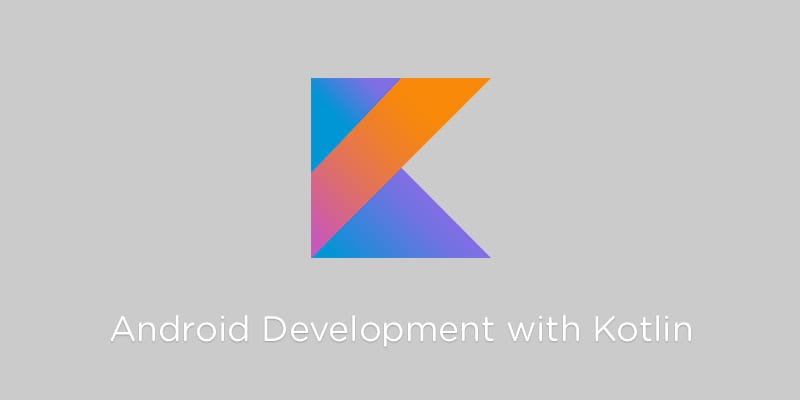
Android Development with Kotlin
It was only a matter of time after Swift came to be in 2014 that Android would decide on a next-generation programming language to push their app development forward. Historically, Java has been considered a complex programming language to learn. If you were a computer science major you were probably force fed Java.
The coming of Swift taught us that there are improvements that could be made in programming languages of yore to improve things like readability, performance, and errors. All of these qualities add up to modern applications that run better and integrate seamlessly into newer frameworks.
In short, Swift cleaned the slate for iOS developers and gave them a new canvas to work with. Last year, Kotlin was released and has tackled a lot of the syntax issues that had made Java somewhat clunky. Google recently added Kotlin over Swift as their main language for future application development.
Note: My back and forth between Swift and Kotlin is not to say that they are similar because they aren’t. Swift is more like Python whereas Kotlin pulls inspiration from languages like Scala and Groovy.
So what’s so good about Kotlin anyway?
It’s about happiness. Consider this function below (taken from Heroku’s Blog Post on Kotlin):
fun main(args: Array<String>) = runBlocking<Unit> {
var number = 0
val random = Random()
val jobs = List(100_000) {
launch(CommonPool) {
delay(10)
number += random.nextInt(100)
}
}
jobs.forEach { it.join() }
println("The answer is: $number")
}
Kotlin, much like Swift, follows the tradition of statically typed languages that are capable of type inference. You will also notice the lack of boilerplate, namely there are no semicolons being used here.
An interesting feature to note here is what Kotlin calls a coroutine. Think of it as a light-weight thread that can run in parallel, wait for and communicate with other threads. What’s so great about them is that they don’t cost much in terms of performance. While there is a coroutine in the code snippet above, let’s take a look at a simple example:
fun main(args: Array<String>) {
println("Start")
// Start a coroutine
launch {
delay(1000)
println("Hello")
}
Thread.sleep(2000) // wait for 2 seconds
println("Stop")
}
The delay() call suspends the coroutine without blocking the thread.
One last small but powerful feature Kotlin implements are how it handles null safety.
val message: String? = null
By adding a ? to the type, the variable becomes nullable. Otherwise, the occurrence of null values could cause runtime errors if one isn’t careful. Null safety removes the risk of such an error from happening.
The Future of Kotlin
On paper, Kotlin appears to have a bright future. Being built inside the Java ecosystem makes it an obvious choice considering its interoperability with Java and how well it works with built tools like Maven and Gradle. It will be exciting to see how it evolves over time as the new champion language for Android development.
You might be wondering where Kotlin falls short? Thankfully there is a great article aptly titled the good, the bad, and the ugly where you can learn about some of Kotlin’s strange design decisions.
- Glide Unveils Groundbreaking AI-Powered Features to Revolutionize No-Code Software Development - August 7, 2023
- How Low-Code Applications Can Help Your Business - August 6, 2023
- Why No-Code Platforms Are Still Relevant in 2023 - August 6, 2023